Introduction to Abstract Syntax Trees in Python
Table of Contents
This article explores Python Abstract Syntax Trees (ASTs). Python developers like that Earthly provides consistent and fast build environments for running Python code. Check it out.
When working with Python, it is essential to have a clear understanding of the code’s structure and how it works. This is where Abstract Syntax Trees (AST) come into play. An AST is a tree-like structure that represents the syntactic structure of a program, making it a powerful tool for Python programmers who want to take their coding skills to the next level. By understanding how Python code is parsed, developers can efficiently analyze thier code leading to more effective and efficient programming.
In this guide, we will introduce you to the concept of AST and provide an overview of its importance in Python programming. We will explore how to use AST for analyzing, transforming, and optimizing your code, as well as provide a real-world example of how AST can be used for static code analysis. By the end of this article, you will have a solid understanding of how to leverage an AST to improve your Python coding skills and create more efficient and effective programs.
Understanding AST
An Abstract Syntax Tree (AST) is a hierarchical, tree-like data structure that represents the syntactic structure of a program. It serves as an intermediate representation of the code and is generated by a compiler or interpreter. The AST captures the essential elements of the program’s syntax, providing a structured representation of operators, functions, statements, and expressions. The AST is constructed by parsing the source code of a program. During the parsing process, the code is analyzed and broken down into its constituent parts, such as keywords, identifiers, literals, and operators. These parts are then organized hierarchically in the form of a tree, with the main program as the root node and the various code elements as its child nodes. The AST effectively captures the structure of the code by representing relationships between different code elements. For example, function calls are represented as child nodes of the corresponding function definitions, and expressions within statements are represented as child nodes of those statements. This hierarchical representation allows for easy traversal and analysis of the code’s structure. To obtain an AST from source code, a parser is used. The parser takes the source code as input and performs lexical analysis and syntactic parsing to identify the different elements and their relationships. The parser follows the grammar rules of the programming language to generate the corresponding AST.
The AST can be thought of as a simplified version of the program’s source code, making it easier to analyze, manipulate, and optimize. This is particularly useful for tools that need to analyze the structure of the program, such as linters, code formatters, and optimization tools. By understanding how to work with ASTs, developers can gain insights into how their code works and identify areas where improvements can be made.
Importance of AST
The importance of Abstract Syntax Trees (AST) in Python programming cannot be overstated. AST serves as a powerful and efficient tool for code analysis and manipulation. By representing the program’s syntax in a structured form, AST empowers developers to perform a wide range of code transformations and optimizations, including bug detection, performance improvement, and memory usage optimization. The significance of AST is particularly evident in the context of Python due to the language’s dynamic nature. Python’s syntax is known for its complexity and flexibility, which can pose challenges when it comes to effectively analyzing and optimizing code. However, AST provides a structured and standardized representation of Python code, offering developers a unified framework to reason about and manipulate the code. This structured approach facilitates the navigation and transformation of Python code, ultimately enhancing the overall quality and efficiency of Python programs.
Some of the way you might use an AST in Python programming (besides parsing) include:
- Code analysis and optimization: AST can be used to analyze the structure of code and identify potential issues such as syntax errors, security vulnerabilities, and performance bottlenecks. It can also be used to optimize code by identifying redundant or inefficient operations.
- Code transformation: AST can be used to transform code by replacing, adding, or removing code elements such as functions, classes, and statements. This is particularly useful for code refactoring, where large codebases need to be updated or modernized.
- Tooling and automation: AST is used extensively in Python tooling and automation, such as linters, code formatters, and static analyzers. These tools use AST to analyze and manipulate code automatically, improving developer productivity and code quality.
Anatomy of an AST Node
Understanding the structure of an AST node is crucial for working with ASTs effectively. Each node in the AST represents a specific element of the program’s syntax, such as functions, statements, and expressions. AST nodes have a defined structure, consisting of a type, attributes, and child nodes.
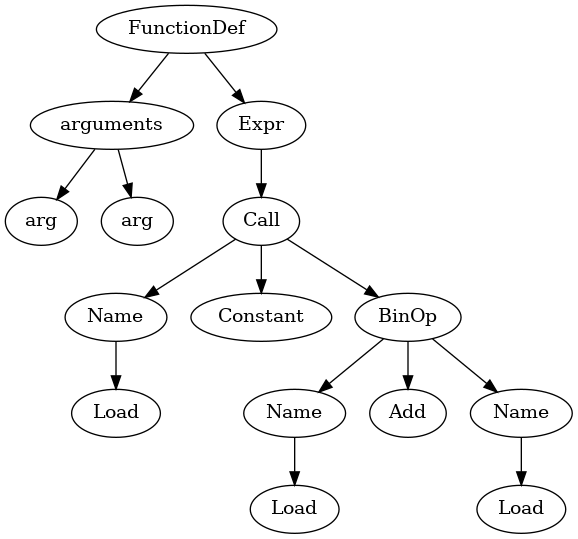
The type of an AST node indicates the kind of syntax element that it represents. For example, a “FunctionDef” node represents a function definition in Python, while an “If” node represents an if statement. Other types of AST nodes include “Assign” for variable assignments, “Call” for function calls, and “Expr” for expression statements.
The attributes of an AST node provide additional information about the node’s type. For example, a “FunctionDef” node will have attributes such as the function name, arguments, and body. An “Assign” node will have attributes such as the variable name and the value being assigned.
The child nodes of an AST node represent the sub-elements of the syntax element it represents. For example, the body of a FunctionDef
node will be a list of child nodes representing the statements that make up the function body.
Here’s an example of an AST node for a simple Python function:
FunctionDef(='my_function',
name=arguments(
args=[arg(arg='arg1'), arg(arg='arg2')],
args=None,
vararg=None,
kwarg=[]
defaults
),=[Expr(value=Num(n=42))],
body=[]
decorator_list )
In this example, the AST node represents a function definition with the name my_function and two arguments. The body of the function contains a single expression statement, which is a number literal with the value 42. The node also has an empty decorator list.
Generating Code with AST in Python
In Python, an AST is generated using the built-in ast
module, which provides a range of functions for parsing Python code and constructing an AST tree. The ast.parse()
function is the primary method for generating an AST tree, which takes a string of Python code as input and returns an AST object that represents the code’s structure.
For example, consider the following Python code:
def greet(name):
print("Hello, " + name + "!")
"John") greet(
To generate an AST tree for this code, we can use the ast.parse()
function as follows:
import ast
= '''
code def greet(name):
print("Hello, " + name + "!")
greet("John")
'''
= ast.parse(code) tree
This will create an AST tree that represents the structure of the code, with each node in the tree corresponding to a specific Python language construct, such as a function definition or a call expression.
We can also use the ast.dump()
function to visualize the AST tree in a more readable format, as follows:
import pprint
pprint.pprint(ast.dump(tree))
This will output the following representation of the AST tree:
("Module(body=[FunctionDef(name='greet', args=arguments(posonlyargs=[], "
"args=[arg(arg='name')], kwonlyargs=[], kw_defaults=[], defaults=[]), "
"body=[Expr(value=Call(func=Name(id='print', ctx=Load()), "
"args=[BinOp(left=BinOp(left=Constant(value='Hello, '), op=Add(), "
"right=Name(id='name', ctx=Load())), op=Add(), right=Constant(value='!'))], "
"keywords=[]))], decorator_list=[]), Expr(value=Call(func=Name(id='greet', "
"ctx=Load()), args=[Constant(value='John')], keywords=[]))], type_ignores=[])")
The output above shows the structure of a program’s syntax in a hierarchical manner. Each element of the code is represented by a node in the AST. In this specific example, the AST represents a Python module that contains a function definition named greet
. The greet
function takes an argument called name
. The body of the function consists of an expression that calls the print
function, passing it a formatted string that includes the value of the “name” argument. To make sense of this AST representation, you can break it down as follows:
The outermost node is the
Module
node, which represents the entire module.Within the
Module
node, there is aFunctionDef
node representing thegreet
function definition.The
FunctionDef
node has various attributes, such as the name of the function (name
) and the arguments it takes (args
).The body of the
greet
function is represented by a list of nodes, in this case, containing a single “Expr” node.The
Expr
node represents an expression, and in this case, it contains a function call toprint
.The function call is represented by the “Call” node, which has the function name (“Name”) as
print
and the arguments it takes.The arguments of the
print
function call include a formatted string that concatenates thename
argument with other strings.
Visualizing the Graph
There are several ways to visualize an AST in Python. One popular library for this purpose is the graphviz
library, which can generate visual representations of graphs and trees. To use graphviz for rendering graphs to PNG, you first need to install it on your system. You can do this by following the installation instructions on the graphviz website for your specific operating system. Once installed, you can then use pip to install the Python bindings for graphviz by running the command pip install graphviz
.
Here’s an example of how to use graphviz
to visualize an AST:
import ast
from graphviz import Digraph
...# Create a Graphviz Digraph object
= Digraph()
dot
# Define a function to recursively add nodes to the Digraph
def add_node(node, parent=None):
= str(node.__class__.__name__)
node_name str(id(node)), node_name)
dot.node(if parent:
str(id(parent)), str(id(node)))
dot.edge(for child in ast.iter_child_nodes(node):
add_node(child, node)
# Add nodes to the Digraph
add_node(tree)
# Render the Digraph as a PNG file
format = 'png'
dot.'my_ast', view=True) dot.render(
In this example, we start by defining a Python code string that contains a simple function. We then use the ast
module to generate an AST from the code. Next, we create a graphviz
Digraph
object to hold the nodes and edges of the AST. We define a recursive function called add_node
to add nodes to the Digraph
, along with their parent nodes and edges. Finally, we call add_node
on the root node of the AST (in this case, the Module
node), and then render the Digraph
as a PNG file after specifying the format and using the render
method. When we open the PNG file, we see a visual representation of the AST, with nodes representing different elements of the code (such as functions, statements, and expressions) and edges representing the relationships between them. By executing the code and rendering the Digraph as a PNG file, a graphical representation of the AST will be generated. The resulting visualization will help visualize the code’s structure, including functions, statements, and expressions, along with their relationships and hierarchy. Opening the generated PNG file (my_ast.png in this case) will display the graphical representation of the AST, allowing for a more intuitive understanding of the code’s structure and organization. Here is an example of how the graph generated by graphviz may look like:
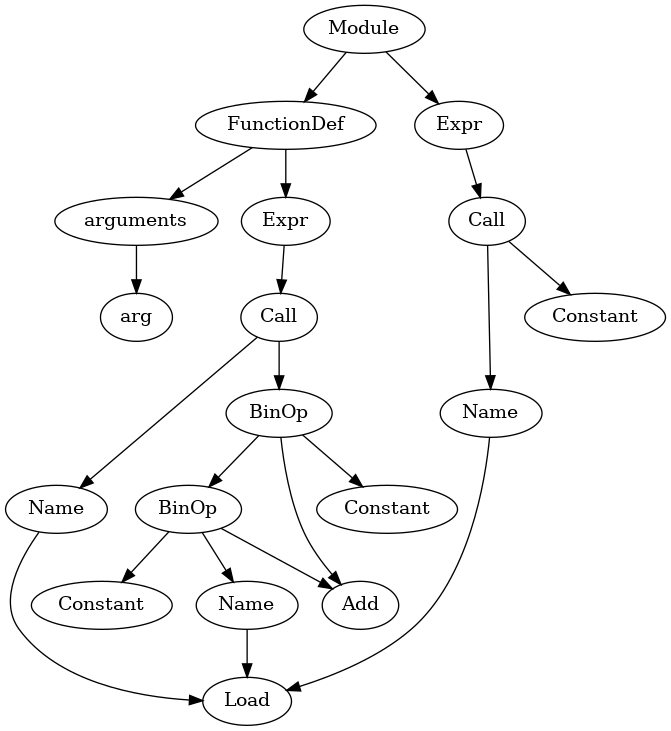
Analyzing Code With AST
Real-World Example: Static Code Analysis
Static code analysis involves analyzing a program’s code without executing it to identify potential issues before deployment. To demonstrate the use of AST for static code analysis, we will use a simple example of a Python program that contains some common coding errors, such as unused variables and functions, as well as code smells that could potentially lead to bugs and security vulnerabilities. We will generate an AST tree from the program’s code and analyze its structure to identify and fix these issues.
By using AST for static code analysis, we can quickly identify and fix potential issues, ensuring that the code is optimized, secure, and bug-free before deployment. This can save developers time and resources by minimizing the need for manual debugging and testing, leading to more efficient and effective programming.
To perform static code analysis on the given code using an AST, we can generate an AST tree and traverse it to identify potential issues. Let’s consider an example where we want to analyze a Python codebase for inefficient string concatenation. We can use the ast.NodeVisitor
class to visit each node in the AST tree and check if any string concatenations are being performed inefficiently. Here’s a suitable example:
import ast
class FunctionCallVisitor(ast.NodeVisitor):
def visit_Call(self, node):
if isinstance(node.func, ast.Name) and node.func.id == "print":
= [arg for arg in node.args if isinstance(arg, ast.Str)]
args if args:
print("Detected print statements with string literals:")
for arg in args:
print(arg.s) # Print the string literal directly
self.generic_visit(node)
The code defines a custom FunctionCallVisitor class that inherits from
ast.NodeVisitor
. This class is used to visit and traverse the nodes in the AST.Inside the FunctionCallVisitor class, there is a method called visit_Call, which is called when a Call node is encountered in the AST. The visit_Call method takes the node as an argument.
The code checks if the Call node represents a function call to the print function by verifying that node.func is an instance of ast.Name and its id attribute is equal to “print”.
If the function call is indeed to print, the code further checks if any of the arguments passed to the print function are string literals by using a list comprehension and checking if each argument is an instance of ast.Str.
If there are string literals among the arguments, the code prints the message “Detected print statements with string literals:” and then iterates over the string literals (arg.s) and prints each one directly.
Finally, the code invokes self.generic_visit(node) to continue visiting other nodes in the AST. This code essentially traverses the AST and detects print statements with string literals, printing those literals directly.
To demonstrate static code analysis using AST in Python, let’s create a function called perform_static_analysis. This function takes a code snippet as input and performs the analysis. First, it parses the code using ast.parse to generate the corresponding Abstract Syntax Tree (AST). Then, it creates an instance of the FunctionCallVisitor class and visits each node in the AST to identify specific function calls. The function call visitor can be customized to detect and analyze specific patterns or behaviors. In our case, we will focus on identifying print statements with string literals. Let’s take a look at the code implementation below:
def perform_static_analysis(code):
= ast.parse(code)
tree = FunctionCallVisitor()
visitor visitor.visit(tree)
The code defines a function called perform_static_analysis that takes the code as an input.
Inside the function, it uses ast.parse(code) to parse the given code and generate an AST (Abstract Syntax Tree) representation of the code.
The resulting AST tree is stored in the tree variable.
It then creates an instance of the FunctionCallVisitor class, named visitor.
Finally, it calls visitor.visit(tree) to start traversing the AST by invoking the visit method of the visitor object and passing the tree as the argument.
This code performs the static code analysis by parsing the given code into an AST and then utilizing the FunctionCallVisitor to traverse the AST and identify print statements with string literals.
Now to observe the output of the code and understand how the static analysis works, let’s consider the following example:
def main():
= '''
code def calculate_average(numbers):
total = sum(numbers)
average = total / len(numbers)
print("Average:", average)
data = [1, 2, 3, 4, 5]
calculate_average(data)
print("End of program")
'''
perform_static_analysis(code)
In this code, we have a main function that defines a multiline string code. The code represents a Python code snippet that calculates the average of a list of numbers and prints the result. The perform_static_analysis function is then called with the code as an argument to analyze the code statically.
Inside the code, there is a function called calculate_average that takes a list of numbers as input. It calculates the total sum of the numbers and then divides it by the length of the list to compute the average. The average value is then printed using the print statement. After defining the calculate_average function, the code snippet creates a list data with some numbers and calls the calculate_average function with data as an argument. Finally, it prints “End of program” to indicate the end of the program execution.
By calling perform_static_analysis(code), the code is analyzed using the FunctionCallVisitor to detect any print statements that use string literals. This analysis helps identify any potential issues or inefficiencies in the code related to printing values. Now when we call our main function, we will see the following output:
main()
Detected print statements with string literals:
Average:
Detected print statements with string literals:
End of program
In conclusion, static code analysis using AST offers developers a powerful tool to analyze their code without executing it. By leveraging the abstract syntax tree representation of the code, developers can detect potential issues before deployment, saving time and resources while ensuring code quality.
Transforming Code With AST
Transforming code with AST involves modifying the structure of the code without changing its behavior. This can be useful for addressing issues related to code smells and technical debt, such as redundant code, unnecessary statements, and outdated syntax. By using AST to transform code, developers can improve code readability, maintainability, and efficiency.
One benefit of using AST for code transformation is that it allows developers to modify code in a precise and controlled manner. For example, instead of searching through the code manually for a specific pattern to replace, developers can use an AST tool to target only the relevant nodes in the code tree. This can save a lot of time and reduce the risk of introducing errors.
To demonstrate the effectiveness of using AST for code transformation, let’s consider a practical scenario. Imagine we have a complex Python codebase consisting of multiple modules and classes. We want to introduce a logging mechanism by adding a logger instance to all relevant functions and methods in the codebase. Manually modifying each function would be tedious and error-prone, especially in a large-scale project. However, by leveraging the power of AST and AST manipulation techniques, we can programmatically traverse the codebase, identify function and method definitions, and automatically inject the necessary logging statements. This approach saves developers significant time and effort while ensuring consistent and reliable modifications across the entire codebase.
class LoggingTransformer(ast.NodeTransformer):
def visit_FunctionDef(self, node):
# Check if the function requires logging
if self.requires_logging(node):
# Create the logger instance
= ast.Assign(
logger_stmt =[ast.Name(id='logger', ctx=ast.Store())],
targets=ast.Call(
value=ast.Attribute(
func=ast.Name(id='logging', ctx=ast.Load()),
value='getLogger',
attr=ast.Load()
ctx
),=[ast.Str(s=node.name)],
args=[]
keywords
),=node.lineno # Set the lineno attribute
lineno
)# Insert the logger instance at the beginning of the function body
0, logger_stmt)
node.body.insert(return node
def requires_logging(self, node):
# Add your logic here to determine if the function requires logging
# For example, you can check function attributes, docstrings,
# or function names
# Return True if logging is required, False otherwise
return True
The code snippet provided defines a class called LoggingTransformer which inherits from ast.NodeTransformer
. This class is responsible for transforming the Abstract Syntax Tree (AST) of a Python code snippet by adding a logger statement at the beginning of each function definition. The visit_FunctionDef method is an override of the default behavior in ast.NodeTransformer
specifically for FunctionDef nodes in the AST. It is called when a FunctionDef node is encountered during the traversal of the AST.
Inside visit_FunctionDef, there is a check to determine if the function requires logging by calling the requires_logging method. This method can be customized according to specific requirements to determine if a function should have logging added to it.
If logging is required, a logger statement is created using an ast.Assign
node. This assigns a new variable logger to the result of the logging.getLogger function call. The targets attribute of the ast.Assign
node specifies the target of the assignment, which is an ast.Name
node representing the variable logger. The value attribute of the ast.Assign
node specifies the value being assigned, which is an ast.Call
node representing the call to logging.getLogger with the function name as an argument. The lineno attribute of the ast.Assign
node is set to the line number of the original function definition to ensure the transformed code maintains accurate line numbers.
Finally, the logger statement is inserted at the beginning of the function body by using the insert method of the node.body list. This effectively adds the logger statement as the first statement in the function.
The requires_logging method is defined to determine if a function requires logging. It can be customized by adding specific logic to check function attributes, docstrings, or function names. In the given example, the method simply returns True, indicating that logging is required for all functions. To actually transform the code and add logging statements, we can use the following approach:
def add_logging(code):
# Parse the code into an AST
= ast.parse(code)
tree
# Transform the AST to add logging
= LoggingTransformer()
transformer = transformer.visit(tree)
transformed_tree
# Convert the transformed AST back to code
= ast.unparse(transformed_tree)
transformed_code
return transformed_code
The add_logging
function takes a code snippet as input and performs the following steps:
Parsing the code into an AST: The ast.parse function is used to parse the code and generate an Abstract Syntax Tree (AST) representation of the code.
Transforming the AST to add logging: An instance of the LoggingTransformer class is created, which is responsible for transforming the AST by adding logging statements. The visit method of the LoggingTransformer is called on the AST, which traverses the tree and applies the necessary transformations.
Converting the transformed AST back to code: The ast.unparse function is used to convert the transformed AST back into code. This function generates Python code from the AST representation.
Returning the transformed code: The transformed code is returned as the result of the
add_logging
function.
Let’s add a useful example to demonstrate the output of the add_logging
function:
def main():
= '''
code def example_function():
# Function body
pass
class ExampleClass:
def __init__(self):
# Constructor body
pass
def example_method(self):
# Method body
pass
'''
= add_logging(code)
modified_code print(modified_code)
The code above demonstrates the usage of the add_logging
function on a specific code snippet. Let’s break it down step by step:
- The main function is defined as the entry point of the program.
- Inside the main function, a multi-line string code is defined, which contains a sample code snippet. This code snippet includes a function named example_function, a class named ExampleClass, and its associated constructor and method.
- The
add_logging
function is called with the code as an argument, which performs the transformation by adding logging statements to the code. - The result of the transformation is stored in the modified_code variable.
- Finally, the modified code is printed to the console using the print function.
Code transformation using Abstract Syntax Trees (AST) offers powerful capabilities for analyzing and modifying code at a structural level. With the help of the ast module in Python, developers can parse code into AST, traverse and manipulate its nodes, and convert it back to executable code. AST-based transformations enable tasks such as static code analysis, code generation, and refactoring. By leveraging AST, developers can gain deeper insights into their code, automate repetitive tasks, and improve code quality and maintainability.
Conclusion
In conclusion, using Python’s abstract syntax trees you can analyze and transform code effectively. It generates a tree representation of a program’s code, which can help identify potential issues and improve code quality and security.
Earthly Cloud: Consistent, Fast Builds, Any CI
Consistent, repeatable builds across all environments. Advanced caching for faster builds. Easy integration with any CI. 6,000 build minutes per month included.